
Assembly language programming allows developers to interact directly with the computer’s CPU, offering fine control over performance and hardware.
For anyone looking to deepen their understanding of computer architecture and low-level programming, assembly projects offer an excellent opportunity to learn how machines execute instructions at the core level.
In this detailed guide, we provide 19+ assembly project ideas for all skill levels, from beginners just getting started to advanced programmers looking to tackle complex system-level challenges.
Research and Use the Format to Select the Best Project to Start With
Beginner-Level Assembly Projects
1. Simple Calculator
Create a program that performs basic arithmetic operations like addition, subtraction, multiplication, and division. The goal is to use the command-line interface to accept user input and provide real-time results.
Skills Gained:
- Arithmetic operations in assembly
- Control flow using conditional jumps
- Register manipulation for storing temporary values Why It’s Important:
Learning how to perform simple calculations teaches the basics of data manipulation and how numbers are stored and processed at the machine level.
2. Temperature Converter (Celsius to Fahrenheit)
Write a program that converts a temperature from Celsius to Fahrenheit and vice versa. Use basic mathematical formulas and display the result based on user input.
Skills Gained:
- Basic arithmetic
- Handling user input and output
- Working with real numbers using floating-point arithmetic Why It’s Important:
This project reinforces how arithmetic operations work in assembly and provides an introduction to data conversion.
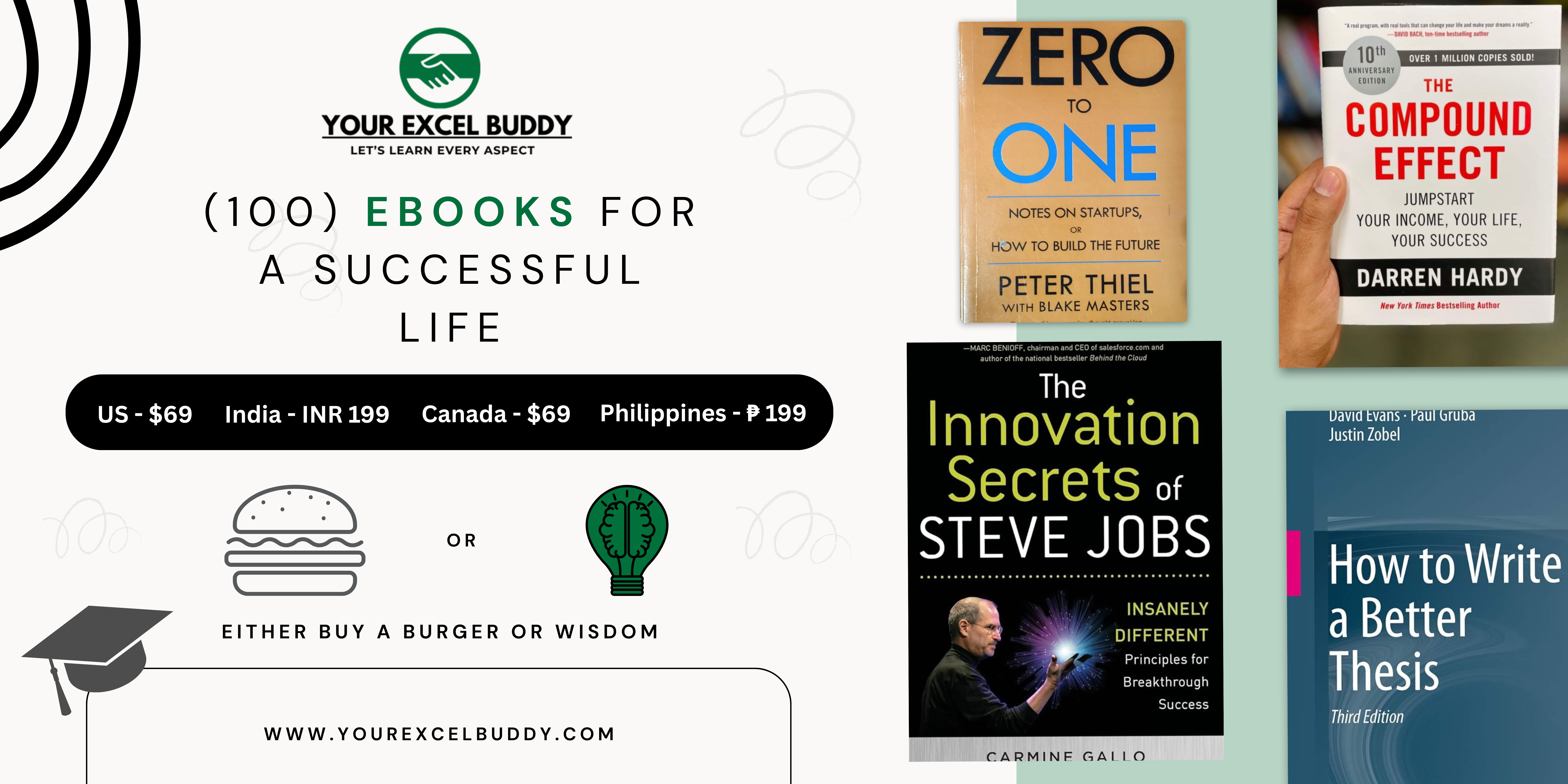
3. Hello World Program
Write a simple assembly program that prints “Hello, World!” to the console. This is the traditional starting point for many programming languages.
Skills Gained:
- Basic output to the screen
- Understanding of the stack and registers
- Learn how to work with interrupts for input/output operations Why It’s Important:
This project gives a basic understanding of how data is sent to the output and teaches you how to handle strings.
4. Odd or Even Number Checker
Create a program that checks if a number entered by the user is odd or even. This requires simple modulus operations and conditional branching.
Skills Gained:
- Conditional branching using jumps
- Bitwise operations for even/odd checks
- User input handling Why It’s Important:
This helps you understand how to control program flow and manipulate bits in a number.
5. Simple Timer
Write a program that acts as a countdown timer. The user can input the time in seconds, and the program will decrement it until reaching zero, at which point it displays a message.
Skills Gained:
- Using loops in assembly
- System clock manipulation
- Real-time data handling Why It’s Important:
Building a timer teaches you how to interface with system resources, such as the CPU clock.
Game Development Project Ideas: Unlocking Creativity for Students
Intermediate-Level Assembly Projects
6. Simple Text Editor
Create a basic text editor that allows users to enter and modify text. The program should enable saving text to a file and reading text from a file.
Skills Gained:
- File handling in assembly
- String manipulation techniques
- User interface design with assembly Why It’s Important:
This project gives you insight into how text editors work and how to manipulate text data at the hardware level.
7. File Encryption/Decryption Program
Develop a file encryption and decryption program that uses basic encryption algorithms like XOR cipher. The program should accept a file and key from the user and encrypt/decrypt the file content.
Skills Gained:
- Cryptographic algorithms in assembly
- File manipulation
- Handling binary data for encryption Why It’s Important:
This project deepens your understanding of data security and file handling.
8. Random Number Generator
Write a program that generates random numbers using seed-based algorithms. You can use this to create a number-guessing game where the computer picks a number, and the user has to guess it.
Skills Gained:
- Pseudo-random number generation
- Algorithm design for randomness
- Memory management for storing values Why It’s Important:
Learning how to generate random numbers in assembly introduces you to algorithmic thinking and data flow management.
9. Prime Number Checker
Build a program that checks if a given number is prime or not. This requires using a loop and division operations to check for factors.
Skills Gained:
- Implementing loops and conditional checks
- Division and modulus operations
- Optimization for performance Why It’s Important:
This project helps you understand how number theory can be implemented in assembly and improves your problem-solving skills.
10. Basic Graphics Renderer
Create a program that renders simple 2D shapes, such as rectangles and circles, to the screen. You will need to interact with the system’s graphics memory and manipulate pixels.
Skills Gained:
- Pixel manipulation and basic graphics rendering
- Working with the video buffer to display graphics
- Optimization for speed and efficiency Why It’s Important:
This project introduces you to the world of graphics programming, where you’ll learn about rendering, memory mapping, and direct hardware access.
11. Assembler Interpreter
Write an interpreter that can take simple assembly-like instructions (such as MOV, ADD, SUB) and translate them into machine code. This project requires parsing user input and simulating assembly commands.
Skills Gained:
- Writing parsers for interpreting input
- Understanding assembly instructions
- Simulation of machine code execution Why It’s Important:
This project enhances your knowledge of how assembly works and gives you a taste of compiler design.
69+ Best Automation Testing Project Ideas to Elevate Your Skills
Advanced-Level Assembly Projects
12. Operating System Shell
Build a basic shell that can accept user commands and execute them. This involves parsing commands, interacting with the file system, and managing processes.
Skills Gained:
- Command parsing
- File management
- Process execution and management Why It’s Important:
This project introduces you to operating system internals, particularly how a shell interacts with system processes and files.
13. Memory Manager
Create a simple memory manager that can allocate and free memory for user processes. This involves managing the heap and stack sections of memory and keeping track of allocated blocks.
Skills Gained:
- Memory allocation techniques
- Heap and stack management
- Optimization for space and speed Why It’s Important:
Understanding memory management is critical for low-level programming, especially in embedded systems.
14. Simple Real-Time Operating System (RTOS)
Develop a real-time operating system kernel with task scheduling and memory management. This project is suitable for embedded systems programmers.
Skills Gained:
- Task scheduling and priority management
- Memory protection techniques
- Handling interrupts in real-time systems Why It’s Important:
Building a real-time OS gives you in-depth knowledge of system internals and how operating systems function.
15. Virtual Machine (VM) Emulator
Write a program that emulates a simple virtual machine capable of running a predefined instruction set. You can implement features such as memory management, registers, and I/O operations.
Skills Gained:
- Instruction set implementation
- Memory and register management
- Handling I/O operations in an emulated environment Why It’s Important:
Building a virtual machine teaches you how high-level languages are translated into low-level operations and how CPUs execute instructions.
16. Game Engine (Basic 2D)
Create a basic 2D game engine in assembly, including a graphics renderer, physics system, and input handling. This project requires advanced knowledge of assembly and hardware interfacing.
Skills Gained:
- 2D graphics rendering
- Physics simulation
- Input device handling for game controllers or keyboards Why It’s Important:
Understanding how to build a game engine helps you learn performance optimization techniques and how real-time systems manage multiple tasks simultaneously.
17. Network Packet Sniffer
Build a program that captures and analyzes network traffic at the packet level. This project involves interacting with network hardware and using low-level network protocols.
Skills Gained:
- Network protocol analysis
- Packet inspection and filtering
- Low-level networking Why It’s Important:
This project gives you a deep understanding of how networks operate and how data is transferred at the packet level.
18. Compiler for Assembly-Like Language
Create a compiler that translates code written in a high-level language into assembly or machine code. This is a complex project that involves parsing, **code generation
**, and *optimization*.
Skills Gained:
- Lexical analysis and syntax parsing
- Code generation in assembly
- Optimization techniques for efficient machine code Why It’s Important:
Writing a compiler gives you a comprehensive understanding of how high-level languages are converted into assembly and machine code.
99+ Design Thinking Project Ideas for Engineering Students to Ignite Innovation
19. Multi-threaded Program
Develop a program that runs multiple threads concurrently, sharing resources and managing synchronization between them. This project deals with thread creation, mutex locks, and semaphores.
Skills Gained:
- Multi-threading and synchronization
- Resource sharing
- Handling concurrency issues such as deadlocks Why It’s Important:
Multi-threaded programming is essential for optimizing system performance, especially in multi-core processors.
Conclusion
Assembly programming may seem challenging, but these 19+ project ideas provide an opportunity to understand how computers operate at the lowest level. Whether you’re just starting or you’re an advanced programmer, each project offers the chance to dive deep into system internals and hardware manipulation.